Avatar
Default avatar
Use this example to create a circle and rounded avatar on an image element.
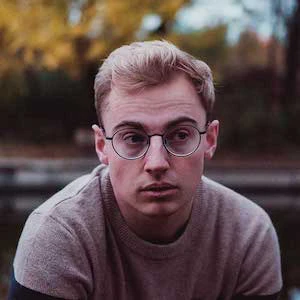
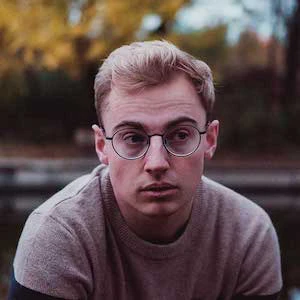
<script>
import { Avatar } from 'flowbite-svelte';
</script>
<Avatar src="/images/profile-picture-2.webp"/>
<Avatar src="/images/profile-picture-2.webp" rounded/>
Bordered
You can apply a border around the avatar component
If you can use the ring-{color} class from Tailwind CSS to modify ring color.
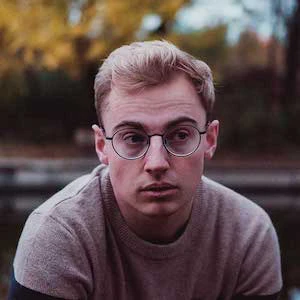
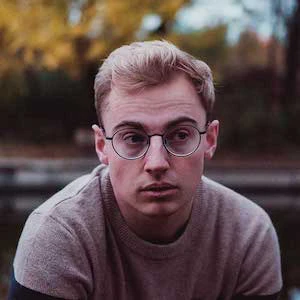
<Avatar src="/images/profile-picture-2.webp" border />
<Avatar src="/images/profile-picture-2.webp" border class="ring-red-400 dark:ring-red-300" />
Placeholder
When there is no custom image available a placehoder is displayed.
<Avatar />
<Avatar rounded />
<Avatar border />
<Avatar rounded border />
Dot indicator
Use a dot element relative to the avatar component as an indicator for the user (eg. online or offline status).
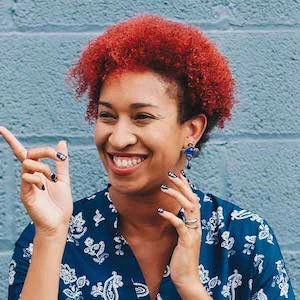
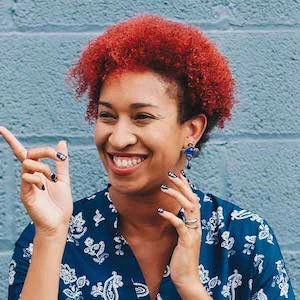
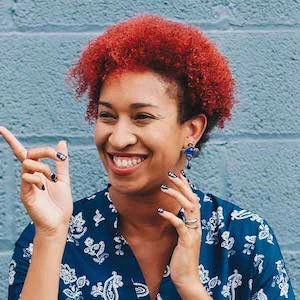
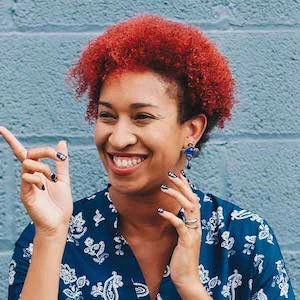
<script>
<Avatar src="/images/profile-picture-3.webp" dot={{top:true, color:"bg-red-400"}}/>
<Avatar src="/images/profile-picture-3.webp" dot={{top:true, color:"bg-red-400"}} rounded />
<Avatar src="/images/profile-picture-3.webp" dot={{color:"bg-green-400"}}/>
<Avatar src="/images/profile-picture-3.webp" dot={{color:"bg-green-400"}} rounded/>
</script>
Stacked
Use this example if you want to stack a group of users by overlapping the avatar components.
<div class="flex mb-5">
<Avatar src="/images/profile-picture-1.webp" stacked />
<Avatar src="/images/profile-picture-2.webp" stacked />
<Avatar src="/images/profile-picture-3.webp" stacked />
<Avatar stacked />
</div>
<div class="flex">
<Avatar src="/images/profile-picture-1.webp" stacked />
<Avatar src="/images/profile-picture-2.webp" stacked />
<Avatar src="/images/profile-picture-3.webp" stacked />
<Avatar stacked href="/">+99</Avatar>
</div>
Avatar text
This example can be used if you want to show additional information in the form of text elements such as the user’s name and join date.
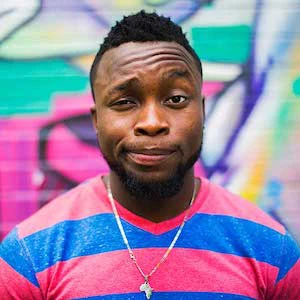
<div class="flex items-center space-x-4">
<Avatar src="/images/profile-picture-1.webp" rounded />
<div class="space-y-1 font-medium dark:text-white">
<div>Jese Leos</div>
<div class="text-sm text-gray-500 dark:text-gray-400">Joined in August 2014</div>
</div>
</div>
User dropdown
Use this example if you want to show a dropdown menu when clicking on the avatar component.
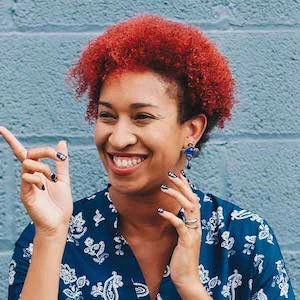
- Dashboard
- Settings
- Earnings
- Sign out
<Dropdown>
<Avatar slot="trigger" src="/images/profile-picture-3.webp" dot={{color:'bg-green-400'}} />
<DropdownHeader>
<span class="block text-sm"> Bonnie Green </span>
<span class="block truncate text-sm font-medium"> name@flowbite.com </span>
</DropdownHeader>
<DropdownItem>Dashboard</DropdownItem>
<DropdownItem>Settings</DropdownItem>
<DropdownItem>Earnings</DropdownItem>
<DropdownDivider />
<DropdownItem>Sign out</DropdownItem>
</Dropdown>
Sizes
Select size from xs | sm | md | lg | xl.
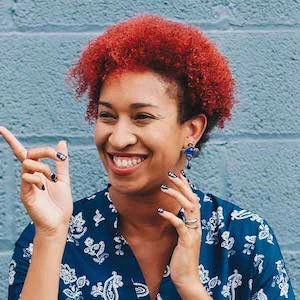
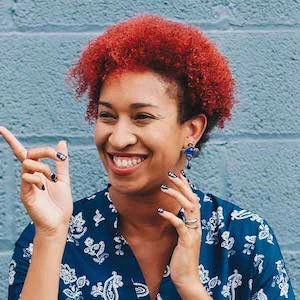
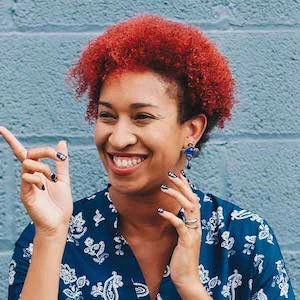
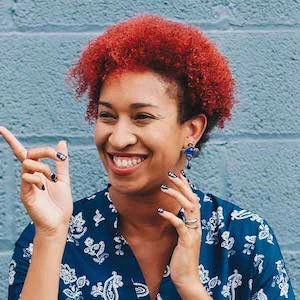
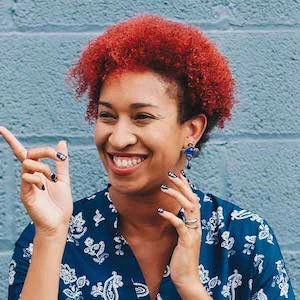
<Avatar src="/images/profile-picture-3.webp" rounded size="xs" />
<Avatar src="/images/profile-picture-3.webp" rounded size="sm" />
<Avatar src="/images/profile-picture-3.webp" rounded size="md" />
<Avatar src="/images/profile-picture-3.webp" rounded size="lg" />
<Avatar src="/images/profile-picture-3.webp" rounded size="xl" />
Props
The component has the following props, type, and default values. See types page for type information.
Name | Type | Default |
---|---|---|
src | string | '' |
href | string | '#' |
rounded | boolean | false |
border | boolean | false |
stacked | boolean | false |
dot | DotType | { top: false, color: 'bg-gray-300 dark:bg-gray-500' } |
alt | string | '' |
size | 'xs' | 'sm' | 'md' | 'lg' | 'xl' | 'md' |